About Course
The Full Stack Web Development Course provides a comprehensive learning experience in building dynamic and responsive web applications. This course covers:
- Front-End Technologies:
- HTML & CSS: Core web markup and styling skills.
- JavaScript: Fundamental programming for interactivity and client-side logic.
- Bootstrap & Tailwind: Modern CSS frameworks for responsive design.
- Sass: Preprocessor for more efficient and organized styling.
- React: Component-based JavaScript library for creating complex user interfaces.
- Back-End Technologies:
- Node.js: JavaScript runtime for server-side development.
- Express: Web application framework for handling requests and building APIs.
- Database Management:
- MongoDB: NoSQL database for handling large volumes of unstructured data.
- CRUD Operations: Techniques for managing data (Create, Read, Update, Delete).
- API Design: Best practices for designing robust APIs for data handling and integration.
- Development Tools:
- VS Code: Code editor with tools and extensions for efficient development.
- Terminal: Command-line usage for executing tasks and managing files.
- Git & GitHub: Version control for tracking changes and collaborating on projects.
This course equips students with a solid foundation in full-stack web development, empowering them to build complete, data-driven web applications and gain skills that are highly valuable in the tech industry.
Course Content
Getting Started
-
Introduction
22:00 -
Roadmap
05:15 -
VS Code Crash Course
01:29:48
Learn HTML
-
Introduction
02:43 -
Creating HTML Files
07:31 -
HTML Elements (Tags)
06:00 -
Document Structure
06:39 -
Headings and Paragraphs
09:38 -
Comments, Line Breaks and WhiteSpaces
08:00 -
HTML Attributes
04:40 -
HTML Images
06:13 -
HTML Links (Anchor Tags)
18:17 -
HTML Lists
09:38 -
HTML Tables
11:23 -
HTML Forms – Part 01
08:12 -
HTML Forms – Part 02
12:00 -
HTML Form Submission
10:16 -
Media – Video and Audio
05:00 -
Divs and Spans
08:00 -
Semantic HTML Elements
04:31 -
Block VS Inline Elements
06:51
Learn CSS
-
Introduction
06:24 -
Inline CSS
06:20 -
Internal CSS
04:34 -
External CSS
05:15 -
CSS Selectors
14:45 -
CSS Colors
25:00 -
CSS Backgrounds
13:49 -
Box Model
04:13 -
Borders
13:24 -
Padding
06:06 -
Margin
05:00 -
Text Formatting
12:48 -
Google Fonts
09:11 -
Icons
10:17 -
Styling Links
07:04 -
Styling Lists
04:24 -
Styling Tables
08:21 -
Transitions
11:04 -
Animations
10:45 -
Flexbox introduction
04:23 -
FlexBox – practical
21:08 -
CSS Grid
18:24 -
SASS
31:02
Learn Bootstrap
-
Introduction of Bootstrap
08:35 -
Setting-up
11:53 -
Typography
15:12 -
Bootstrap Colors
07:22 -
Bootstrap Buttons
12:57 -
Utility Classes
21:27 -
Bootstrap Container VS Container Fluid
07:00 -
Bootstrap Grid
13:57 -
Images
03:56 -
Bootstrap tables
06:42
Project – Fully Responsive Website
-
Project Introduction
02:47 -
Project Files and Resources
-
Navbar
23:52 -
Hero Section
14:30 -
Newsletter Section
08:09 -
Popular Courses Section
09:09 -
Popular Courses Section – Part 2
22:08 -
Pricing Plan Section
17:14 -
Learn Section
11:16 -
FAQ Section
07:11 -
Contact Information
10:09 -
Footer Section
04:24 -
Bootstrap Modal and Forms
10:46
Learn Tailwindcss – Latest Update (tailwind v4)
-
Introduction
10:00 -
How Does Tailwind Works
07:48 -
Setting Up Tailwind
09:14 -
Tailwind Colors
15:55 -
Margin and Padding
16:51 -
Tailwind Typography
32:19 -
Sizing
13:24 -
Layout and Position
13:45 -
Backgrounds and Shadows
19:32 -
Borders
13:20 -
Filters
19:46 -
Interactivity
27:00 -
Responsive Design – Breakpoints
12:18 -
Columns
10:05 -
Tailwind Flexbox
25:37 -
Tailwind Grid
12:27 -
Transform
11:53 -
Tailwind Transitions
10:02 -
Tailwind Animations
12:33
Tailwind Project
-
Project Setup
05:00 -
Creating Navigation
15:21 -
Creating a Hero Section
05:48 -
Instructors and Learners Section
10:20 -
Recent Courses Section
21:38 -
View All Courses Card
03:33 -
Courses Page
11:31 -
Course Details Page
31:31 -
Add Course Page
27:13
Learn JavaScript
-
Introduction
21:49 -
Setting up JavaScript
08:00 -
JavaScript Code Files
00:00 -
Console
14:08 -
JavaScript Comments
05:27 -
Variables – Theory
09:47 -
Variables – Practical
11:34 -
Data Types
13:40 -
Type Conversion
10:42 -
JavaScript Operators – Theory
18:39 -
JavaScript Operators – Practical
16:57 -
Strings
21:00 -
Math Object
07:27 -
Dates
02:27 -
Arrays
08:24 -
Array Methods
11:55 -
Array Nesting, concatenation and spread
12:59 -
Objects
11:36 -
Destructuring
10:15 -
JSON
13:00 -
Basics of Functions
14:27 -
More on Function Parameters
08:22 -
Function Expression
03:00 -
Arrow Function
05:00 -
IIFE( Immediately Invoked Function Expression
03:10 -
If statements
00:00 -
If else statement
06:37 -
Else if and Nested If
11:30 -
Switch Statement
07:45 -
Ternary Operator
04:49 -
For Loop
09:40 -
Break and Continue
04:50 -
While and Do While Loop
07:11 -
For Of Loop
05:35 -
For in loop
04:41 -
Array.Foreach Method
06:00 -
Array.map method
07:28
Learn DOM (Document Object Model)
-
Introduction
07:00 -
Selecting DOM Elements
21:52 -
Changing the content of Elements and Attribute Values
14:57 -
Changing the style of element
09:00 -
Creating New Attributes
09:15 -
Events and Event Listerners
13:00 -
ClassLists (Add, Remove, and Toggle)
11:15 -
Creating New HTML Elements
08:33 -
Navigating Between DOM Elements
11:15
JS Project 1 – Responsive Menu (Hamburger Menu)
-
Project Intro
01:10 -
HTML Part
06:33 -
CSS Part
23:00 -
JavaScript Part
11:00
JS Project 2 – Sidebar Project
-
Project Introduction
02:00 -
HTML Part
09:25 -
CSS Part
21:19 -
JavaScript Part
08:28
JS Project 3 – Counter Application
-
Project Intro
01:06 -
HTML Part
03:46 -
CSS Part
08:17 -
JavaScript Part
12:48
JS Project 4 – Notes Taking Application
-
Project Intro
02:19 -
HTML and CSS Part – Layout
21:30 -
Javascript Part 1 – Adding, removing and editing notes
19:19 -
JavaScript Part 2 – Saving Notes To Local Storage
17:53
JS Project 5 – Animated Login Form
-
Project Introduction
01:00 -
HTML and CSS Part
17:53 -
JavaScript Part
12:00
JS Project 6 – Password Length Checker
-
Project Intro
01:00 -
HTML and CSS Part
17:15 -
JavaScript Part
12:00
JS Project 7 – Text To Speech Convertor
-
Project Intro
01:14 -
HTML & CSS Text To Speech App
16:16 -
JavaScript Part 1 – Convert text to speech
11:00 -
JavaScript Part 2 – Add Voices
12:12 -
JavaScript Part 3 – Adding Pause and Resume
08:00
JS Project 8 – Random User Generator
-
Project Introduction
01:00 -
HTML and CSS
10:41 -
JavaScript Part
24:14
Learn Git & GitHub
-
Introduction
10:24 -
Downloading and Installing Git
05:00 -
Git configuration
02:33 -
Basic Windows Commands
13:24 -
Git Workflow
16:38 -
Undo changes – Part 1 – Working Area
04:34 -
Unstaging files
05:51 -
Reset Commit
06:44 -
Git Ignore (.gitignore)
03:56 -
Git Branching
21:10 -
Merge Conflict
06:41 -
Use VSCode instead of terminal – GUI
07:08 -
Creating github account
08:47 -
First GitHub Repository + Local Repository
15:10 -
Cloning Remote Git Repository
04:18 -
Git Push, Pull and Fetch
07:47 -
Pull Request and Merge
09:38
Learn React
-
Introduction
10:00 -
Prerequisites and Tools
06:27 -
Downloading and Installing Node.js
11:00 -
Customizing Windows Terminal (Optional)
21:00 -
Creating First React App – Using CRA
10:02 -
Creating React App – Using Vite
06:53 -
VSCode Integrated Terminal
06:05 -
React Folder Structure
14:45 -
Let’s Clear Files
08:25 -
Creating React Component
10:42 -
Challege – Components
13:00 -
JSX and Its Rules
08:15 -
Expressions in JSX
05:14 -
Challege – JSX Expressions
10:43 -
Rendering a List
12:43 -
List Challenge
08:08 -
Destructuring
03:15 -
Props
09:17 -
Props Challenge
08:18 -
Children Props
04:47 -
Condtional Rendering
10:00 -
Challenge – Conditional Rendering
19:19 -
Styling components
15:30 -
Bootstrap + React
05:29 -
Tailwind + React
07:00 -
Daisy UI
10:09 -
FlowBite React
08:51 -
React Icons
03:25 -
Events
09:53
Iftiinshe Academy -React Project
-
Project Setup
06:12 -
Adding Tailwind and Git
07:14 -
Downloading Theme Files
03:10 -
Starting Home Page
08:42 -
NavBar Component
06:28 -
Hero Component
06:25 -
Cards Wrapper Component
10:12 -
Course Listings and Course Listing Component
06:40 -
Rendering Courses Info from JSON File
09:34 -
Limit Courses to only 3
03:26 -
Toggle Description – useState Hook
09:00 -
Adding Icon – React Icons
04:18 -
React Router Setup
17:00 -
Link Component
12:00 -
Not-Found Page
07:19 -
Active Links
08:00 -
Courses Page
08:13 -
Introduction about API
06:06 -
JSON Server
05:39 -
Data Fetching From an API – useEffect
14:49 -
Loading – React Spinner
16:29 -
Creating Single Course Details Page
07:58 -
Rendering Single Course Info
-
Getting Course ID from the URL – useParams
08:36 -
Add Course Page Component
04:47 -
React Form Handling
22:00 -
Saving the course – POST Request
-
useNavigate
04:42 -
Deleting Course – DELETE Request
11:00 -
Adding confirmation before course deletion
-
React Toast
06:52 -
Edit Course Page
09:33
Learn MongoDB
-
Downloading MongoDB, MongoDB Compass, and MongoDB Shell
08:29 -
Collections and Documents
03:34 -
Creating Database, Collection and Document using MongoDB Compass
13:00 -
Using MongoDb Shell
09:32 -
Ways to insert Documents into collections
10:30 -
Finding Data From Collections
13:19 -
Limit, Sort and Skip
06:47 -
Deleting Documents
02:04 -
Updating Documents
04:05 -
Filtering Documents
17:00
Learn Node & Express
-
Getting Started Node.js
07:32 -
Basics of the Node.js
12:05 -
Creating Node Modules
04:24 -
Node Packages and NPM
10:31 -
HTTP Server
06:22 -
Creating Express Server
08:27 -
Creating Get Routes and End Points
13:27 -
Route Parameters
13:36 -
Post Request
23:00 -
PUT Request
19:35 -
Patch Request
19:20 -
Delete Request
07:34 -
Middlewares
23:14 -
Express Validator
25:00 -
Validation Schema
09:00 -
Code Refactoring Part 1- Routes
14:25 -
Code Refactoring Part – 02 Controls and Middlewares
16:28
Mongoose – Node + MongoDB + Mongoose + Express
-
Understanding Mongoose
03:00 -
Installing Mongoose and Connecting to MongoDB
08:00 -
Creating Schema
07:00 -
Creating a Model
05:48 -
Saving Documents (Data)
06:10 -
Querying (Getting) Documents
15:00 -
Using Mongo & Mongoose Comparison Operators
08:00 -
Logical Operators
04:20 -
Updating Documents (Data)
15:00 -
Deleting Documents (Data)
04:44 -
Schema Validation
05:53 -
More on validations
05:00
Full-Stack Project – 01 – Book Store
We'll use in this project:
1. React
2. Tailwind
3. Zustand
4. Node
5. Express
6. MongoDB
7. Git and GitHub
8. Railway - For Deployment
-
Project Intro
08:50 -
Source Code
-
Installed Express and Setup Express Server
07:00 -
Installing and Configuring Nodemon
09:00 -
Creating Boilerplate API Routes
12:38 -
Connecting to MongoDB
11:00 -
Creating The Book Model
07:40 -
Creating Save Book API Endpoint
15:27 -
Code Refactoring
15:00 -
Creating Get All Books API Endpoint
05:12 -
Creating API Endpoint for Getting Single Book By ID
07:31 -
Creating Update Book API End-Point
08:18 -
Creating Delete Book API End-Point
04:33 -
Installing React and Tailwind
09:42 -
Creating NavBar Component
15:26 -
Adding and Configuring React Router
08:00 -
Getting Books From The API
11:00 -
Installing and Configuring Zustand
10:40 -
BookCard Component
26:44 -
Adding Heading To The Home Page
07:00 -
Designing Add New Book Page
08:35 -
Completing Add New Book Functionality
27:39 -
Completing Delete Book Functionality
10:27 -
Adding Confirmation Dialog Box
20:40 -
Designing Update/Update Book Modal
20:00 -
Completing Update Book Functionality
27:00 -
Building Book Details Page
24:00 -
Configuring Proxy
03:00 -
Preparing The Project For Deployment
20:00 -
Deployment
13:00
Full-Stack Project – 02 – Car Marketplace (Car Listing Site)
-
Project Intro
06:25 -
Setting up
10:10 -
Pages and Routes
10:32 -
Header UI
15:27 -
Express Server
07:39 -
Configuring Environment Variables and Nodemon
07:00 -
Connecting to MongoDB Atlas
12:39 -
User Model
06:21 -
First Test API Route
09:50 -
Signup API Route
28:00 -
Error Handler Middleware
07:26 -
Signup Page UI
12:10 -
Signup Page Functionality
19:00 -
Toast Notifications
14:00 -
Signing API Route
24:00 -
Signing Page Functionality
08:45 -
AppContext For State Management
18:00 -
Save Logged in user date into the Local Storage
06:44 -
OAuth Button
06:07 -
OAuth Functionality
34:54 -
Update Header
09:00 -
Protect Profile Page
09:39 -
Profile Page UI
12:50 -
Upload Profile Image Functionality
30:00 -
Update User API Route
23:00 -
Update User Functionality
23:54 -
Toast Notification for Updates
07:10 -
Delete User Functionality
17:56 -
Sign-out User Functionality
14:37 -
Car Listing Model
15:08 -
Listing API Route
13:00 -
Listing Page UI
37:59 -
Upload Listing Images Functionality
37:19 -
Completing Create Listing Page Functionalities
42:00 -
Fix 01
07:00 -
Get User Listings – API Route
21:00 -
Show User Listing
23:00 -
Deleting Listing
14:11 -
Update Listing API Route
09:43 -
Get Listing Data By ID
04:44 -
Creating Listing Page
18:18 -
Adding Image Slider
24:00 -
Completing Listing Page
38:00 -
Fix 02
07:11 -
Seller Contact Functionalities
31:55 -
Search API Route
30:00 -
Adding Some Search Functionalities in the header
11:00 -
Search Page UI
18:00 -
Search Page Functionalities 01
56:00 -
List Item Component
49:00 -
Search Result Pagination
11:31 -
Home Page
29:00 -
About Page
15:29 -
Preparing For Deployment
14:00 -
Render Deployment
20:23
AI – Project – ChatBot
-
11:13
-
Setting up the project and installing tailwindcss
10:17 -
Creating the user interface
20:35 -
Getting Gemini API and Adding to your Application
09:50 -
Creating and Sending First Prompt
08:32 -
Saving Previous Prompts into Message History
21:18 -
Display Chats
15:48 -
Finishing UP
13:00
Earn a certificate
Add this certificate to your resume to demonstrate your skills & increase your chances of getting noticed.
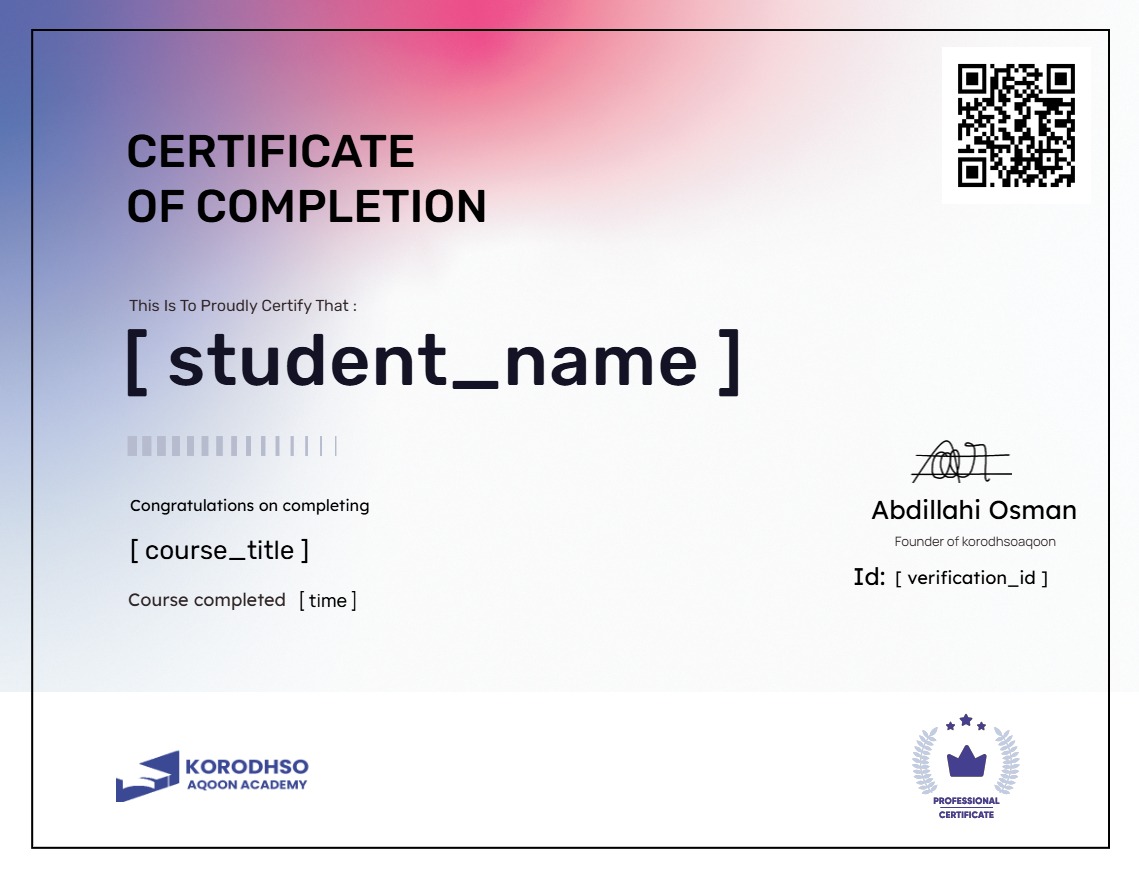